Mastering React Hooks: A Practical Guide
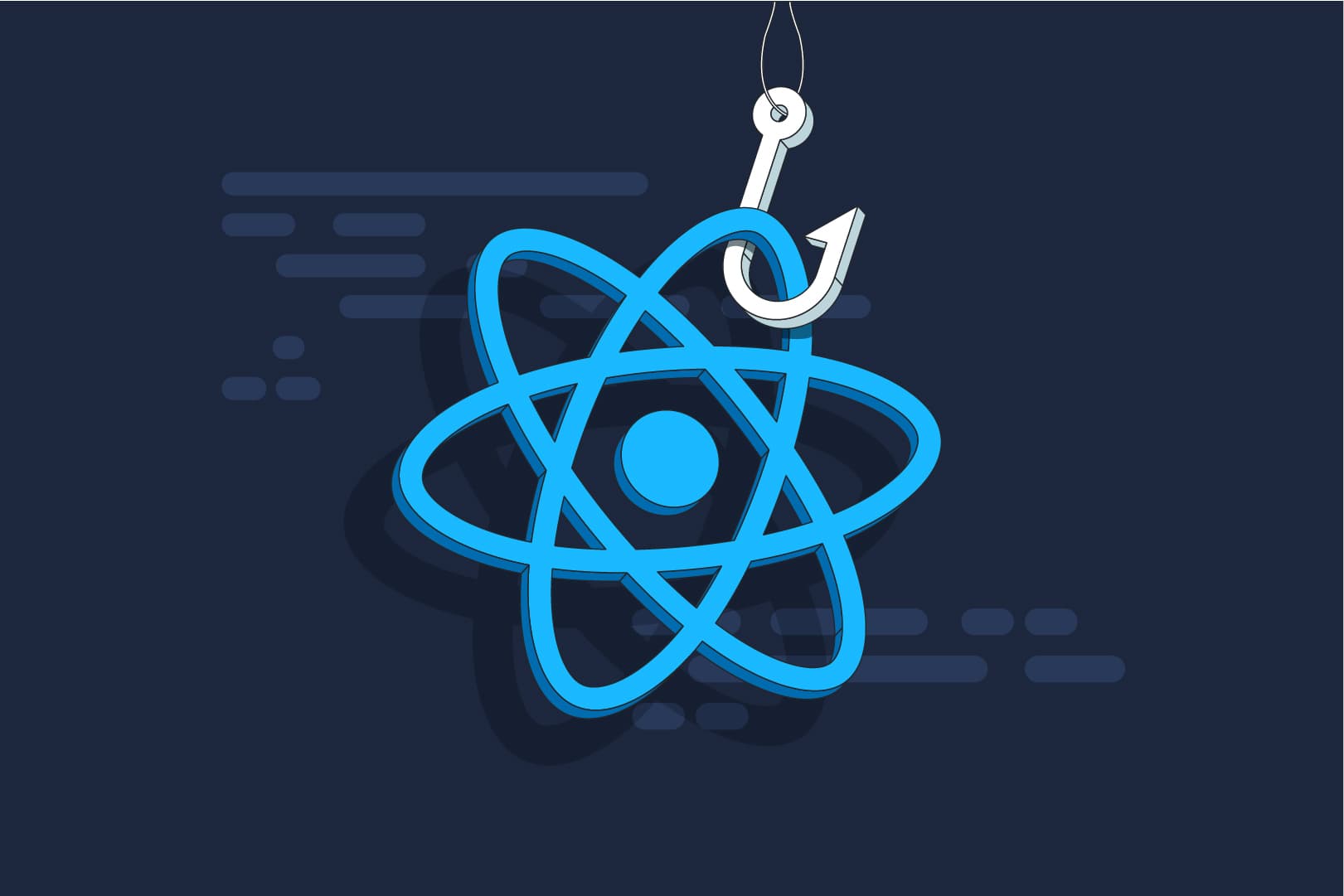
React Hooks revolutionized how we write React components by allowing us to use state and other React features in functional components. This guide provides a practical overview of essential React Hooks, complete with examples to help you master them.
Why Use Hooks?
Before Hooks, state management and lifecycle methods were only available in class components. Hooks solve this by letting you "hook into" React state and lifecycle features from functional components, making your code cleaner, more reusable, and easier to understand.
Essential React Hooks
useState: Managing State
The useState
Hook lets you add state to functional components. It returns an array containing the current state value and a function to update that value.
import { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
useEffect: Handling Side Effects
The useEffect
Hook lets you perform side effects in functional components. Side effects are operations that affect something outside the component, such as data fetching, subscriptions, or manually changing the DOM.
import { useState, useEffect } from 'react';
function DataFetcher() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch('/api/data');
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const json = await response.json();
setData(json);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchData();
}, []);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
if (!data) return null;
return (
<div>{/* Display data */}</div>
);
}
useContext: Accessing Context
The useContext
Hook lets you access context values. Context provides a way to pass data through the component tree without prop drilling.
import { useContext } from 'react';
import MyContext from './MyContext'; // Your context
function MyComponent() {
const value = useContext(MyContext);
return <p>Value from context: {value}</p>;
}
Other Important Hooks
useReducer
: For complex state logic.useCallback
: For memoizing functions.useMemo
: For memoizing expensive calculations.useRef
: For accessing DOM elements.
This guide covers the most essential React Hooks. Exploring the official React documentation is highly recommended for a deeper understanding.